Sending Emails Using Python and Gmail
Introduction
Sending personal emails using Python is less secure but it is also sometimes valuable for certain use cases. Gmail used to allow the user to send emails using the Gmail account and the password directly in Python. However, this method has been removed recently because of its severe security issues.
In this blog post, I would like to quickly discuss how to send emails using Python and Gmail nowadays.
Python Gmail Authentication
Previous Authentication
Previously, we could just use the Gmail account and the password for the Gmail SMTP login in Python when “Allow less secure apps to ON” is enabled and the Google account 2-step verification disabled for the Google account. However, it is extremely unsecure because whenever someone got the password they could easily change it and the user would lose the account forever. Even if the Google account is “disposable” for the user, sometimes it is confusing to the Gmail recipients that the Gmail sender address got changed due to the previous one was stolen.
New Authentication - More Secure But More Complicated
From May 30, 2022, no “Allow less secure apps to ON” is allowed for any Google account. Therefore, the previous Gmail authentication method in Python will not work anymore.
Google encouraged the user to use the Gmail API for managing the emails. However, the authentication setup process and verification process are complicated. Credential setups are saved in the application system environment, the application is deployed in a new environment, manual authentication process will be required. This prevented the application from being deployed on the cloud.
New Authentication - Less Secure But Less Complicated
There is, however, another hidden way for Gmail authentication in Python, which is setting up an App password while the Google account 2-step verification is enabled. App passwords let you sign in to your Google Account from apps on devices that don’t support 2-Step Verification. It is less secure. That’s probably why Google did not advocate it. However, it is less secure in a sense that someone could use your Gmail account to send emails on your behalf if they happen to get your App password, but they could not change your Google account password if they don’t know the it. The Google account owner could change or delete the App password anytime if they found the Gmail account has been compromised.
After setting up the 2-step verification and App password for Google account, the Google account security might look like this.
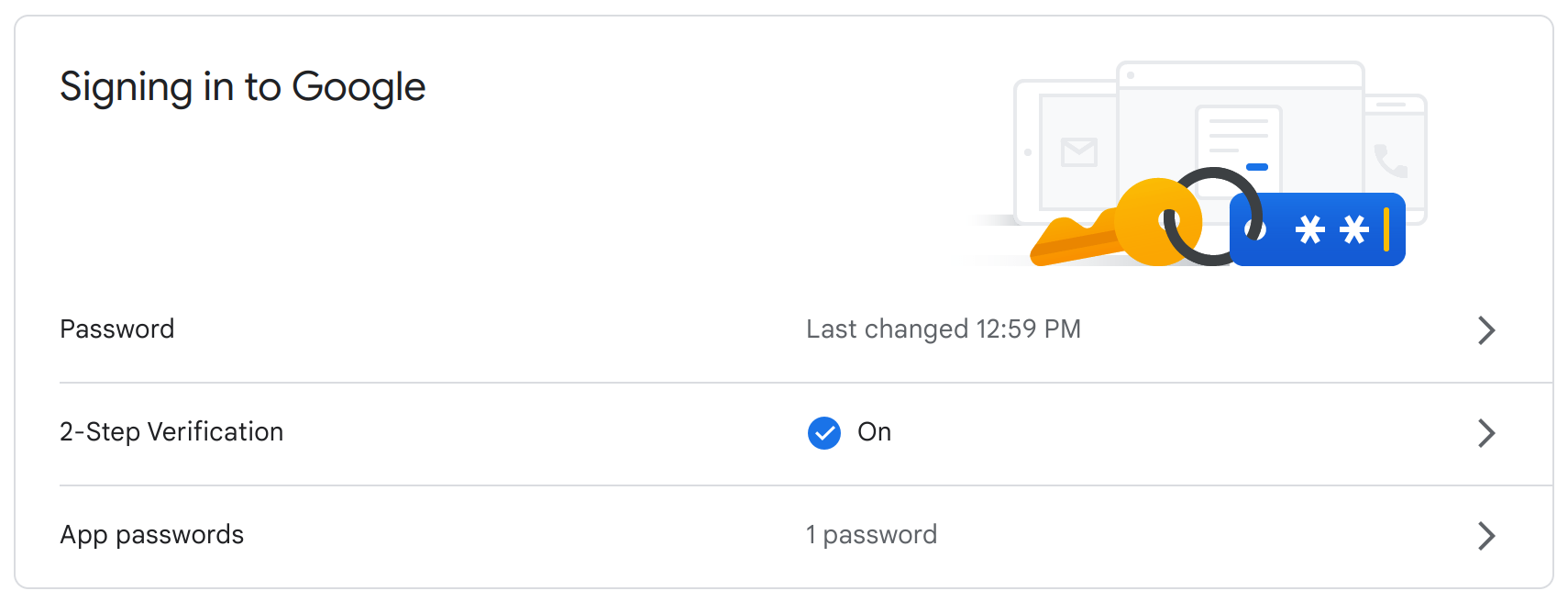
Python Sending Gmail
In the Python script, instead of using the Google account password, we use the App password for Gmail SMTP authentication.
1 | import smtplib, ssl |
The reader is encouraged to try the above Python script.
Sending Emails Using Python and Gmail